Have you ever stared at a tangled mess of code, wondering how you’ll ever manage to add a new feature without breaking everything? Or perhaps you’re building a complex system and struggling to keep the different parts organized and communicating effectively? This is where Domain-Driven Design (DDD) comes in. It’s a powerful methodology designed to help developers create software that closely reflects the business domain it’s meant to serve.
Image: github.com
GoLang, with its clean syntax, strong typing, and robust standard library, is a fantastic language for implementing DDD principles. This article will be your guide to understanding and implementing DDD in GoLang, covering everything from basic concepts to practical examples and helpful online resources.
Understanding the Domain-Driven Design Approach
What is Domain-Driven Design?
Domain-Driven Design is a software development approach that emphasizes a deep understanding of the business domain. It’s a collaborative process that involves both developers and domain experts, working together to build a shared understanding of the problem space and how the software should model it. Instead of focusing solely on technical details, DDD prioritizes business logic and how the software should solve real-world problems.
Why Use Domain-Driven Design?
DDD offers several benefits:
- Improved Code Organization: DDD helps you structure your code around meaningful business concepts, making it easier to understand and maintain.
- Enhanced Communication: By providing a common language for developers and business stakeholders, DDD facilitates smoother collaboration and avoids misunderstandings.
- Increased Scalability: DDD promotes modularity and reusability, enabling you to build systems that can adapt to changing business requirements.
- Reduced Complexity: DDD helps you break down complex domains into smaller, more manageable pieces, making the development process less intimidating.
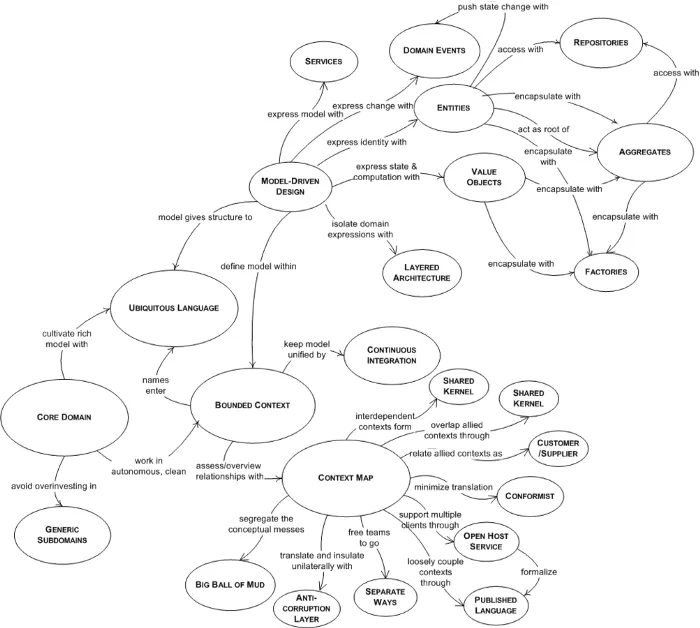
Image: programmingpercy.tech
Core Concepts in Domain-Driven Design
Ubiquitous Language
The principle of “ubiquitous language” is crucial to DDD’s success. This refers to creating a shared vocabulary for describing the domain, understood by both developers and business stakeholders. Using consistent terminology ensures everyone is on the same page, reducing ambiguity and misunderstandings. For example, instead of using vague terms like “item” or “order,” agree on specific terms like “Product” and “Purchase Order.”
Bounded Contexts
Bounded contexts are like autonomous islands within your domain. Each context represents a specific part of the business, with its own unique set of rules, models, and language. Maintaining clear boundaries between contexts helps to prevent unwanted dependencies and ensure each part of the system can evolve independently.
Aggregates
An aggregate is a cluster of related objects that are treated as a single unit. This ensures data consistency—when you update one part of the aggregate, the others are updated accordingly. It also simplifies interaction with the domain, as you work with the aggregate as a whole, rather than individual objects.
Implementing Domain-Driven Design with GoLang
Structuring your GoLang Project
When implementing DDD in GoLang, it’s helpful to structure your project in a way that reflects the bounded contexts. Consider creating separate packages for each context, with each package containing its own models, repositories, and services. For instance, you might have a “Customer” package, an “Order” package, and a “Payment” package.
Using Go Interfaces
Go interfaces are essential for creating flexible and maintainable code. Use interfaces to define the behavior of your domain entities, allowing you to switch between different implementations without affecting other parts of your application. This promotes loose coupling and makes it easier to adapt your code to future changes.
Leveraging Go’s Concurrency Features
Go’s support for concurrency (goroutines and channels) is a natural fit for implementing complex domain scenarios. Use concurrency to effectively handle asynchronous operations, like sending notifications or processing background tasks, while maintaining a clean and concise code base.
Using Go’s Testing Framework
Don’t underestimate the importance of testing in DDD. Write comprehensive tests for your domain objects and services, focusing on both unit testing and integration testing to ensure the correctness and viability of your logic within the wider system.
Real-World Examples
Let’s look at a concrete example of how you might implement DDD in a GoLang application:
Imagine you’re building an e-commerce platform. You might establish the following bounded contexts:
- Customer Management: This context handles user accounts, addresses, and other customer-related information.
- Order Processing: This context manages the creation, fulfillment, and shipment of orders.
- Inventory Management: This context tracks stock levels, product availability, and other inventory related data.
- Payment Processing: This context handles payment processing, including integration with third-party payment gateways.
Each context would have its own entities, repositories, and services. For example, the “Order” context might have an “Order” entity, an “OrderRepository” interface, and an “OrderProcessor” service. These would be part of the “Order” package within your Go project.
Online Resources for Learning DDD with GoLang
If you’re ready to delve deeper into Domain-Driven Design with GoLang, here are some fantastic online resources:
Blogs and Articles
- Domain Driven Design with Go by Eric Evans: This blog post provides a comprehensive introduction to DDD concepts with GoLang examples.
- Domain Driven Design in Go – Part 1 by James Golick: This article focuses on specific implementation techniques for DDD with GoLang.
- Domain Driven Design with Go (DDD Go) by DDD Go: This blog offers insights into practical applications of DDD principles in Go projects.
Books
- Domain-Driven Design: Tackling Complexity in the Heart of Software by Eric Evans: This classic book is the definitive guide to DDD, providing a comprehensive overview of the methodology.
- Implementing Domain-Driven Design by Vaughn Vernon: This book provides practical guidance on implementing DDD principles in real-world projects.
Courses
- Domain-Driven Design in Go with Real-World Examples by Udemy: This course teaches you everything you need to know to apply DDD to GoLang projects effectively.
- Domain-Driven Design (DDD) Masterclass by Pluralsight: This course covers DDD concepts in depth, focusing on their practical implementation.
Read Domain-Driven Design With Golang Online
Conclusion
Domain-Driven Design with GoLang allows you to create software that is robust, maintainable, and scalable. By focusing on the business domain, you can build systems that are genuinely valuable and meet the evolving needs of your users. With the help of online resources, you can embark on a journey of mastering DDD and transforming your GoLang projects for the better.